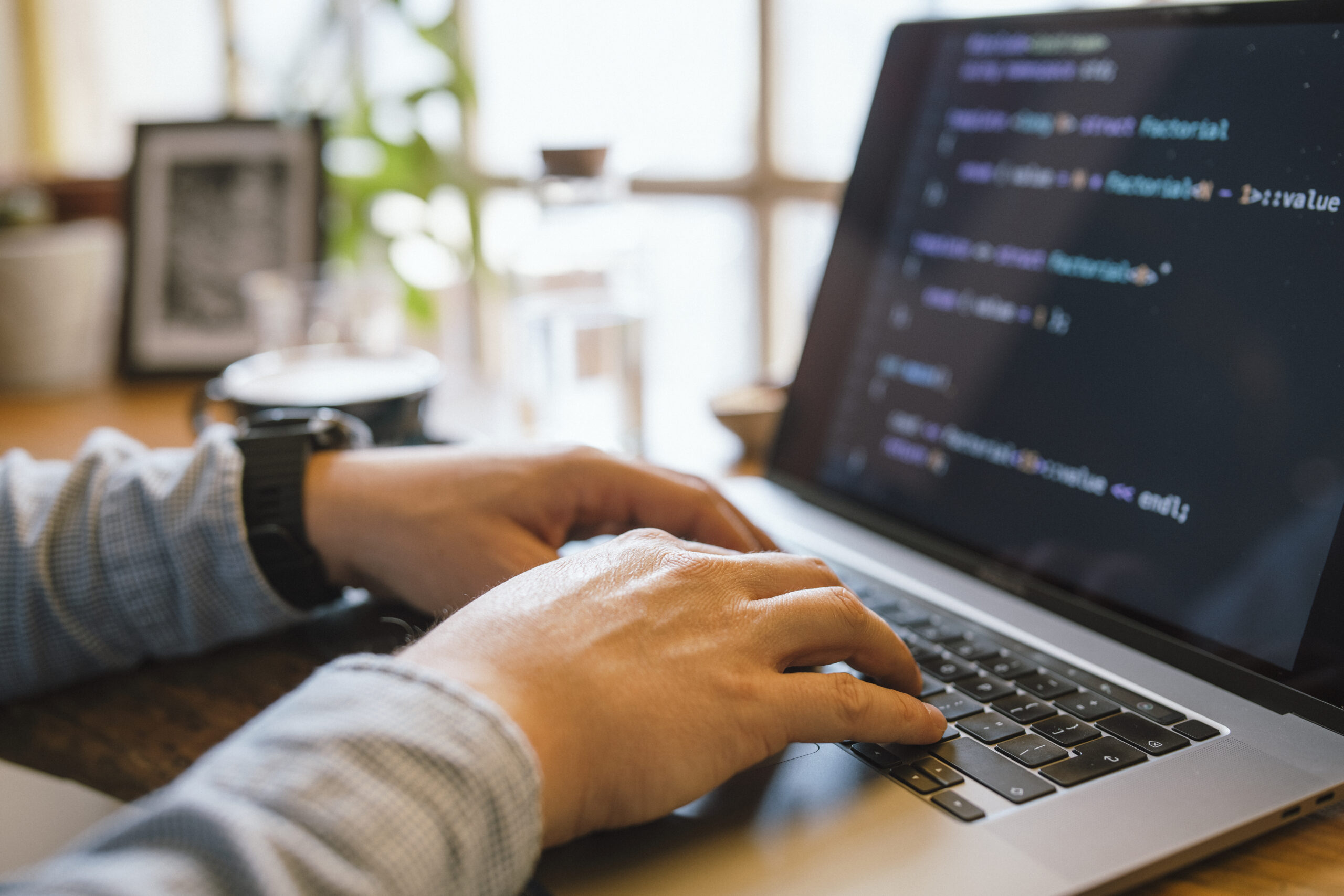
Debugging is One of the more essential — nevertheless generally missed — abilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go wrong, and learning to Believe methodically to solve issues effectively. Regardless of whether you're a newbie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and substantially increase your productiveness. Listed below are numerous methods to assist builders amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Resources
Among the quickest means builders can elevate their debugging expertise is by mastering the resources they use every day. Though producing code is one particular Portion of advancement, understanding how to connect with it properly in the course of execution is equally significant. Present day improvement environments occur Outfitted with potent debugging abilities — but several builders only scratch the floor of what these tools can perform.
Consider, such as, an Integrated Development Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications let you established breakpoints, inspect the value of variables at runtime, move by code line by line, and even modify code around the fly. When applied effectively, they Permit you to notice accurately how your code behaves for the duration of execution, and that is priceless for tracking down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for entrance-finish developers. They help you inspect the DOM, keep track of community requests, view true-time overall performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can convert irritating UI difficulties into workable duties.
For backend or process-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of functioning processes and memory management. Mastering these applications might have a steeper Finding out curve but pays off when debugging general performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, come to be comfy with Edition Management devices like Git to understand code background, locate the exact minute bugs ended up released, and isolate problematic variations.
Ultimately, mastering your tools implies heading over and above default options and shortcuts — it’s about establishing an personal familiarity with your progress ecosystem to make sure that when issues arise, you’re not lost in the dark. The better you understand your equipment, the more time you can spend resolving the particular challenge in lieu of fumbling by the procedure.
Reproduce the challenge
Among the most essential — and sometimes disregarded — actions in efficient debugging is reproducing the problem. Right before leaping to the code or producing guesses, developers have to have to produce a regular surroundings or scenario where by the bug reliably seems. Devoid of reproducibility, repairing a bug becomes a activity of probability, usually leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting just as much context as is possible. Request questions like: What steps led to The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it gets to be to isolate the precise situations less than which the bug happens.
When you’ve gathered sufficient facts, make an effort to recreate the condition in your local ecosystem. This could signify inputting the identical details, simulating equivalent person interactions, or mimicking method states. If The problem seems intermittently, contemplate producing automated exams that replicate the edge situations or point out transitions involved. These assessments don't just aid expose the situation but also avert regressions Down the road.
Occasionally, The difficulty may be surroundings-precise — it might take place only on specified functioning systems, browsers, or below distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the issue isn’t only a phase — it’s a way of thinking. It requires patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be now halfway to fixing it. With a reproducible scenario, You can utilize your debugging equipment additional proficiently, exam opportunity fixes properly, and connect additional Evidently with all your workforce or people. It turns an summary grievance into a concrete challenge — and that’s where builders prosper.
Examine and Fully grasp the Error Messages
Error messages tend to be the most respected clues a developer has when something goes wrong. Rather than looking at them as discouraging interruptions, developers must discover to treat mistake messages as immediate communications from your program. They frequently let you know exactly what transpired, wherever it occurred, and occasionally even why it transpired — if you understand how to interpret them.
Commence by reading the information meticulously and in whole. A lot of developers, specially when beneath time pressure, look at the initial line and immediately start out producing assumptions. But further while in the error stack or logs may well lie the correct root cause. Don’t just duplicate and paste error messages into search engines — read through and comprehend them initially.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Does it issue to a particular file and line selection? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally follow predictable designs, and Discovering to recognize these can substantially increase your debugging procedure.
Some faults are vague or generic, and in All those cases, it’s vital to look at the context in which the error transpired. Test related log entries, input values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger problems and provide hints about probable bugs.
Ultimately, error messages are usually not your enemies—they’re your guides. Understanding to interpret them effectively turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a more productive and self-confident developer.
Use Logging Sensibly
Logging is one of the most strong instruments in a very developer’s debugging toolkit. When utilized properly, it offers authentic-time insights into how an software behaves, serving to you have an understanding of what’s going on under the hood without needing to pause execution or phase from the code line by line.
A fantastic logging system starts off with realizing what to log and at what degree. Frequent logging stages include things like DEBUG, Facts, WARN, ERROR, and Lethal. Use DEBUG for in-depth diagnostic information throughout improvement, Details for normal situations (like thriving start-ups), WARN for potential concerns that don’t split the appliance, ERROR for real issues, and Deadly once the method can’t carry on.
Steer clear of flooding your logs with too much or irrelevant details. An excessive amount logging can obscure significant messages and decelerate your technique. Center on vital situations, condition alterations, enter/output values, and critical read more conclusion factors within your code.
Format your log messages Plainly and constantly. Consist of context, like timestamps, ask for IDs, and function names, so it’s easier to trace difficulties in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Throughout debugging, logs Permit you to observe how variables evolve, what circumstances are achieved, and what branches of logic are executed—all without having halting the program. They’re Primarily worthwhile in production environments wherever stepping by means of code isn’t possible.
Moreover, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Eventually, intelligent logging is about stability and clarity. Which has a effectively-assumed-out logging strategy, you can decrease the time it's going to take to spot troubles, attain deeper visibility into your purposes, and improve the Over-all maintainability and reliability of the code.
Think Like a Detective
Debugging is not simply a technological task—it's a sort of investigation. To correctly identify and resolve bugs, developers ought to solution the process like a detective fixing a thriller. This mindset aids stop working complex problems into manageable elements and comply with clues logically to uncover the foundation bring about.
Get started by gathering proof. Look at the symptoms of the issue: error messages, incorrect output, or functionality difficulties. Identical to a detective surveys against the law scene, collect as much related details as it is possible to devoid of leaping to conclusions. Use logs, examination situations, and consumer studies to piece collectively a transparent photo of what’s occurring.
Up coming, type hypotheses. Request your self: What might be creating this behavior? Have any changes recently been built into the codebase? Has this challenge transpired just before below similar instances? The intention will be to slim down prospects and determine opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the trouble within a controlled setting. Should you suspect a specific purpose or element, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, ask your code issues and Allow the effects direct you closer to the reality.
Shell out close attention to smaller specifics. Bugs frequently hide from the minimum envisioned spots—like a lacking semicolon, an off-by-one error, or a race affliction. Be thorough and client, resisting the urge to patch the issue devoid of totally knowledge it. Short-term fixes may well hide the true problem, only for it to resurface afterwards.
Lastly, hold notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging approach can help you save time for potential difficulties and help Other folks have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical skills, strategy complications methodically, and turn out to be simpler at uncovering concealed challenges in complex techniques.
Produce Checks
Writing exams is one of the best solutions to improve your debugging abilities and All round growth performance. Checks don't just help catch bugs early but additionally serve as a safety net that gives you self-assurance when generating improvements towards your codebase. A perfectly-analyzed software is much easier to debug mainly because it helps you to pinpoint exactly exactly where and when an issue occurs.
Start with device checks, which deal with unique capabilities or modules. These smaller, isolated checks can promptly expose irrespective of whether a selected bit of logic is Doing work as anticipated. Whenever a check fails, you instantly know where to search, substantially decreasing the time used debugging. Device assessments are Specifically beneficial for catching regression bugs—problems that reappear after Beforehand currently being set.
Next, combine integration assessments and finish-to-end checks into your workflow. These support make certain that numerous aspects of your software function alongside one another efficiently. They’re specifically useful for catching bugs that come about in sophisticated systems with many elements or services interacting. If a thing breaks, your exams can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing exams also forces you to definitely Consider critically about your code. To check a feature adequately, you will need to be familiar with its inputs, anticipated outputs, and edge conditions. This amount of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, crafting a failing examination that reproduces the bug is often a powerful initial step. As soon as the check fails persistently, you can give attention to correcting the bug and watch your examination go when The difficulty is resolved. This technique makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, writing exams turns debugging from the disheartening guessing sport into a structured and predictable approach—encouraging you catch much more bugs, more rapidly plus more reliably.
Take Breaks
When debugging a tricky concern, it’s uncomplicated to be immersed in the problem—looking at your display for hrs, hoping Alternative after Answer. But Just about the most underrated debugging equipment is actually stepping absent. Getting breaks will help you reset your head, lower irritation, and infrequently see The difficulty from the new point of view.
When you are far too near to the code for far too very long, cognitive tiredness sets in. You would possibly start out overlooking evident problems or misreading code that you just wrote just hrs previously. Within this state, your Mind results in being fewer economical at challenge-fixing. A short walk, a espresso split, or even switching to another undertaking for ten–15 minutes can refresh your concentrate. Many builders report acquiring the basis of an issue when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Specifically during for a longer period debugging periods. Sitting before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping away means that you can return with renewed Strength along with a clearer mentality. You could possibly abruptly see a lacking semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
Should you’re trapped, a superb rule of thumb is usually to set a timer—debug actively for 45–sixty minutes, then take a five–10 moment crack. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily below limited deadlines, however it essentially results in speedier and more effective debugging Eventually.
In short, using breaks is not really a sign of weak point—it’s a sensible technique. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging is often a mental puzzle, and rest is an element of resolving it.
Discover From Just about every Bug
Every bug you come across is much more than simply A short lived setback—It is a chance to improve as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or maybe a deep architectural issue, each one can educate you anything precious if you make an effort to mirror and examine what went Erroneous.
Start out by inquiring yourself a few important queries after the bug is solved: What induced it? Why did it go unnoticed? Could it are caught before with superior tactics like device tests, code opinions, or logging? The responses generally expose blind places with your workflow or knowledge and make it easier to Make more robust coding practices transferring forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or popular faults—which you could proactively stay away from.
In group environments, sharing what you've acquired from the bug using your peers can be Primarily highly effective. No matter whether it’s through a Slack information, a short create-up, or A fast expertise-sharing session, assisting Many others stay away from the exact same difficulty boosts staff efficiency and cultivates a stronger Discovering lifestyle.
More importantly, viewing bugs as classes shifts your state of mind from frustration to curiosity. In place of dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not the ones who produce ideal code, but individuals that constantly master from their blunders.
Eventually, Every bug you deal with adds a whole new layer to your ability established. So next time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Summary
Bettering your debugging techniques takes time, follow, and tolerance — but the payoff is big. It would make you a far more effective, assured, and able developer. Another time you're knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.